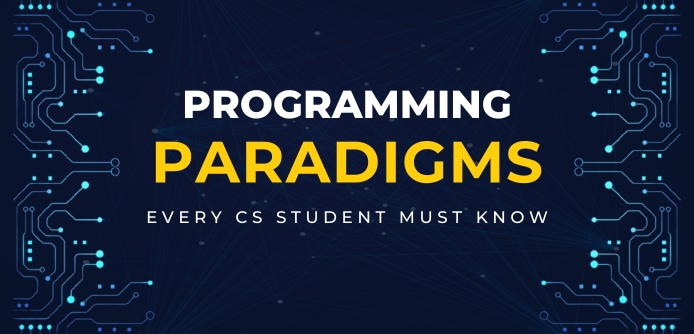
In real life, when we encounter some problem, we analyze it, make processes, and take steps to solve that specific problem. While doing so, we directly communicate and order our mind to take actions according to the processes. At the end, we come up with the problem’s solution. In the same way the programming works. A computer itself is a dumb machine. It cannot think on its own and even cannot take actions towards problem-solving. Programming is the process of providing instructions to computers to perform specific tasks through algorithms. When we try to solve our specific real-time problem using computer, we communicate and order the computer to take specific actions to solve that particular problem. In order to communicate with the computers, we need help of programming languages which serve as a bridge between human-readable code and machine-executable instructions.
Now programming has different paradigms. A programming paradigm is generally a style that guides programmer with the process of writing code. Understanding various programming paradigms is essential for every computer science (CS) student, as these paradigms provides a structured approach towards problem-solving. In this blog, we will explore programming paradigms, categorize them into Imperative and Declarative Programming, and delve into examples using JavaScript.
Let’s start by understanding the concept of programming.
What is the programming?
Programming is the art and science of instructing computers to perform specific tasks using algorithms. It involves creating a set of instructions for the computer. These set of instructions is known as the code. The computer executes this code and performs a specific task (which we want to be performed).
Think of it as you want to cook an egg for your loved one in the breakfast. What exactly will you do? Definitely you will take actions to do so because it’s impossible to cook an egg without taking proper actions. In actual, you will instruct your mind with the step-by-step tasks or procedures to cook an egg. Your mind will work accordingly and at the end you will come up with the cooked egg. Like you may instruct your mind as:
- Take an egg.
- Break the egg shell and take out the egg white and yolk in a bowl.
- Stir it continuously till the egg white and the yolk are mixed up together evenly.
- Add some spices and mix them in the mixture.
- Then take a pan. Take enough oil in the pan to cook the egg.
- Pour the mixture into the pan with heated oil and cook it.
The computer has its own specific language of understanding the instructions (code). And that language is known as the programming language. The computer does not understand the language of humans. It only understands the language of programming. This programming language serves as a bridge between human and computer. It enables programmers to communicate with computers effectively.
Suppose you speak English and you are meeting with the French person for the first time in life and you don’t know how to speak French. There will be a communication barrier. Now to continue the communication, you need a person with you who knows both the English and the French. You will talk to that person in English, he then will translate your message to the other person in French, and his answer will be translated to you by the same middle man in English. Now as we know, the computer does not understand the language of humans. So, to communicate with the computer we need a language which computer understands and that is called as a programming language. This serves as a middle entity between a human and a computer.
So, the human instructs computer through programming and uses a specific programming language to instruct. Now this also involves the paradigms of programming. Let’s explore these programming paradigms.
What is meant by the programming paradigm?
A programming paradigm is a methodology or style that guides the process of writing code. It encompasses principles and practices that dictate how code should be structured, how data should be organized, and how algorithms should be designed. There are multiple ways of solving a specific problem. Every human mind thinks differently and it acts uniquely when it comes to solving a specific problem.
Let’s take an example to understand this perspective. A cooking competition was held between three master chefs and all of three chefs were assigned with the same task of cooking the vegetable rice. Now each chef cooked the vegetable rice and when the results are presented to judges, the judges came up with the point that each chef cooked the vegetable rice differently and with unique taste while ingredients provided to all of them were same in quality and quantity. Here question arises that if ingredients were all same then why the end result of all of the chefs are different in taste. This is because the process of utilizing the ingredients of each chef and the process of cooking the vegetable rice of each chef was different. Each chef came up with the unique and different cooking processes and the end results of all of them were same with different in taste and quality. In the same way, if we give a same problem to three expert programmers and we ask them to solve it, each programmer will solve it differently. Here programming paradigms actually helps the programmer to take actions effectively towards problem-solving. A programmer cannot take blunt decisions while solving a problem same as a chef cannot take blunt decisions while cooking.
We all know that the multiple ways of cooking the rice are taught to a chef while his training period. In the same way, there are multiple ways and styles of programming which are taught to a programmer. That’s why each person who is a programmer must have knowledge of the different programming paradigms so that he/she can take wise decisions when it comes to problem-solving. Selecting a paradigm (way or style of writing the code) totally depends on the nature of the problem. Same problem can be solved by utilizing different paradigms. It’s the programmer’s decision that by which paradigm he/she wants to solve the problem. Following that paradigm, the programmer designs algorithm and then convert it into the code.
For CS students, understanding programming paradigms is crucial as it provides a roadmap for effective problem-solving. Two overarching categories are Imperative and Declarative Programming. Let's delve deeper into each, exploring their subcategories and providing examples in JavaScript.
What are the major categories of programming paradigms?
Programming paradigms can be broadly categorized into two types: Imperative and Declarative. Both of these types come up with the multiple sub-categories. Let’s explore all of them:
1. Imperative Programming Paradigm
Imperative programming is a programming paradigm that focuses on describing a sequence of steps to be executed by the computer to achieve a specific goal. It is characterized by the use of statements that change a program's state. In imperative programming, the emphasis is on "how" to achieve a result, and the programmer explicitly details the control flow and manipulates the program's state. The major sub-categories of imperative programming paradigm are as follows:
1.1. Procedural Programming Paradigm:
Procedural programming is a subset of imperative programming paradigm which focuses on breaking down a program or organizing the code into procedures or routines. In procedural programming, the code is executed step-by-step. The line of code at first will be executed first and then the other whole lines of code will be executed step-by-step. The key features of procedural programming paradigm are as follows:
Key Features of Procedural Programming Paradigm:
1) Procedures and Routines:
- Programs are organized into smaller, manageable procedures or steps to be executed.
- Each procedure encapsulates a set of instructions related to a specific task.
2) Sequential Execution:
- Execution proceeds in a linear and sequential fashioned.
- Statements are executed one after another, following the order specified in the code.
3) State Modification:
- Procedures often manipulate the program's state through the use of variables.
- Variables hold data that can be modified during program execution.
Let’s understand the procedural programming paradigm concept with an example:
- function calculateArea(length, width) {
- let area = length * width;
- return area;
- }
This example represents a simple procedural function, calculateArea, which takes two parameters, length and width. The function defined here acts as a procedure which calculates the area by multiplying these two parameters and then returns the answer. The focus is on the step-by-step execution of instructions. All the code lines will be executed one by one and this is characteristic of the procedural programming paradigm, where the emphasis is on defining procedures or routines that are executed sequentially.
1.2. Structured Programming Paradigm:
Structured programming is another facet of the imperative programming paradigm that emphasizes the use of structured control flow to enhance code readability and maintainability. In structured programming, the problem is solved or execution takes place in a control structure. This structure basically controls the program execution in a mannered way. As a programmer, we can use any control structure like conditional statements or looping structures. Both of these programming fundamentals represent the control structures and if we try to solve a problem or a piece of problem while utilizing these control structures then it means that we are using the structured programming paradigm. The key features of structured programming paradigm are as follows:
Key Features of Structured Programming Paradigm:
1) Structured Control Flow:
- Utilizes constructs like loops and conditionals to create well-defined structures.
- Enhances code readability and makes it easier to understand and maintain.
2) Modularity:
- Encourages breaking down the program into smaller, reusable modules.
- Modules can be procedures or functions that perform specific tasks.
Let’s understand the structured programming paradigm concept with an example:
- if (condition) {
- // code block
- } else {
- // code block
- }
In this example, a conditional statement (if-else) is used to control the flow of the program based on a specified condition. This is an illustration of structured programming, a subset of imperative programming. Structured programming aims to enhance code readability and maintainability by organizing code into well-defined structures. Here, the structured control flow is achieved using the if-else construct.
1.3. Object Oriented Programming (OOP) Paradigm:
Object oriented programming (OOP) is an extension of the imperative programming paradigm that introduces the concept of objects, encapsulation, inheritance, and polymorphism. It promotes modular code. Many programmers like to do programming by making the modules of functionalities. This programming paradigm helps them a lot in making the modules and to pursue modules based programming due to its unique and strong features. The key features of object oriented programming paradigm are as follows:
Key Features of Object Oriented Programming (OOP) Paradigm:
1) Objects:
- Programs are structured around objects, which represent real-world entities.
- Objects encapsulate data and behavior within a single unit.
2) Encapsulation:
- Data and methods that operate on the data are encapsulated within an object.
- Encapsulation promotes data hiding and abstraction.
3) Inheritance:
- Classes can inherit properties and behaviors from other classes.
- Facilitates code reusability and promotes a hierarchical organization of code.
4) Polymorphism:
- Objects of different types can be treated as objects of a common base type.
- Enables flexibility and extensibility in the code.
Let’s understand the object oriented programming paradigm concept with an example:
- class Circle {
- constructor(radius) {
- this.radius = radius;
- }
- calculateArea() {
- return 3.14 * this.radius * this.radius;
- }
- }
- let myCircle = new Circle(5);
- console.log(myCircle.calculateArea());
This example demonstrates the Object Oriented Programming (OOP) Paradigm. The class Circle encapsulates data (radius) and behavior (calculating area) within a single unit. Objects (instances of the class) can be created to represent individual circles. OOP emphasizes the concept of objects, encapsulation, inheritance, and polymorphism, promoting modular and reusable code. This code of class Circle can be referred as a module for calculating the area of a circle.
2. Declarative Programming Paradigm
Declarative programming is a programming paradigm that emphasizes expressing the logic of a computation without explicitly describing its control flow. In declarative programming, the programmer specifies "what" result the program should achieve instead of focusing on "how" the program will achieve a result, leaving the underlying implementation details abstract. This paradigm contrasts with the imperative style, where the focus is on describing the process of achieving a result. The major sub-categories of declarative programming paradigm are as follows:
2.1. Functional Programming Paradigm:
Functional programming is a subset of the declarative programming paradigm that treats computation as the evaluation of mathematical functions. It avoids changing state and mutable data. It emphasizes immutability and the use of higher-order functions. When we try to solve the program using the functions, it means we are utilizing the functional programming paradigm. If we have the processes and the steps or instructions to solve the problem and we analyze that these steps should be executed in a functional way and we create a function to execute these steps, then it means we are utilizing functional programming paradigm. The key features of functional programming paradigm are as follows:
Key Features of Functional Programming Paradigm:
1) Pure Functions:
- Functions in functional programming are pure, meaning they produce the same result for the same input and have no side effects.
- Pure functions contribute to predictability and ease of reasoning about the code.
2) Immutability:
- Emphasizes immutability, discouraging the modification of data once it is created.
- Immutability simplifies the understanding of code and helps prevent bugs related to share mutable state.
3) Higher-Order Functions:
- Functions can take other functions as arguments or return them as results.
- Enables the creation of more abstract and reusable code.
Let’s understand the functional programming paradigm concept with an example:
- const square = (x) => x * x;
- console.log(square(4));
In this example, a pure function square is defined using arrow function syntax. The function takes a parameter x and calculates its square. Functional programming emphasizes the evaluation of mathematical functions without side effects. This function is pure because it consistently produces the same result for the same input, and it doesn't modify any external state.
2.2. Logical Programming Paradigm:
Logical programming is a declarative programming paradigm based on formal logic. Programs consist of logical statements that describe relationships and constraints. A logical programming paradigm revolves around the concept of a logic program, which is essentially a collection of sentences expressed in logical form. These sentences serve to encapsulate knowledge pertaining to a specific problem domain. The essence of computation in the logical programming paradigm lies in the application of logical reasoning to the information encoded in the logical sentences.
The strength of the logical programming paradigm lies in its ability to perform computation through logical inference. Rather than prescribing a sequence of steps for computation, as seen in imperative paradigms, logical programming focuses on declarative statements and allows the system to deduce solutions based on logical relationships.
It could be hard to understand the actual concept of Logical Programming Paradigm. So, let’s understand the logical programming in a simpler way with some real world example.
A simplified example of logical programming paradigm:
Imagine you have a magical book that helps you solve puzzles. In this book, you write down facts, rules, and questions in a special language. This magical book is like a logical program.
- Facts are things you already know. For example, "The sky is blue" or "Birds can fly." These are like your base knowledge.
- Rules are instructions that tell you how things are connected. For example, "If it's raining, take an umbrella" or "If it's a bird, it can sing." These rules help you make decisions.
- Questions are what you want to find out. You might ask, "Is it going to rain today?" or "Can this bird sing?" These questions guide your exploration.
Now, when you want to solve a problem, you open your magical book and let it do the thinking. It uses logical reasoning to connect facts and follow rules to answer your questions.
Example:
Let's say you write:
- Fact: "Sparrows are birds."
- Rule: "If it's a bird, it can sing."
Now, you can ask: "Can sparrows sing?" The logical program looks at the fact and applies the rule to give you the answer: "Yes, sparrows can sing!"
So, in logical programming, you're not telling the computer exactly what to do. Instead, you're giving it knowledge (facts and rules) and asking it questions. The computer uses logic to figure out the answers based on what it knows.
Real-World Comparison:
Think of a detective solving a mystery. The detective has clues (facts), knows some rules about how things work, and asks questions to solve the case. Logical programming is like being a detective with a magical book of knowledge.
So, logical programming is like having a conversation with the computer using facts, rules, and questions. It's a powerful way to solve problems, especially when you want the computer to figure things out on its own!
The key features of logical programming paradigm are as follows:
Key Features of Logical Programming Paradigm:
1) Logic Rules:
- Programs are defined by a set of logical rules and facts.
- Logical programming languages, such as Prolog, use these rules to infer answers to queries.
2) Declarative Knowledge Representation:
- Knowledge is represented declaratively, stating what is known rather than how to find it.
- Suitable for problem domains with a natural declarative representation.
Let’s understand the logical programming paradigm concept with an example of code:
- // Prolog-style example in JavaScript
- function ancestor(X, Y) {
- parent(X, Y);
- }
- function ancestor(X, Y) {
- parent(X, Z);
- ancestor(Z, Y);
- }
This example attempts to simulate a logical programming scenario. In logical programming, programs are based on formal logic, and relationships are expressed through logical statements. The function ancestor expresses a logical relationship, stating that if X is the parent of Y, then X is also an ancestor of Y. This mirrors the style of logical programming languages like Prolog.
Now as we have learnt about the major programming paradigms, understanding their importance and roles in programming is also important. So, let’s delve into it.
Why paradigms are important in programming?
The role of paradigms in programming is fundamental in guiding the design, structure, and approach to writing computer programs. Programming paradigms provide a set of principles and concepts that shape how programmers think about and organize code. Understanding their role is crucial for creating effective, maintainable, and scalable software. Let's explore why programming paradigms are important:
1) Abstraction:
- Definition: Abstraction involves hiding complex details and exposing only essential features.
- Role: Programming paradigms offer abstraction mechanisms, allowing programmers to focus on high-level concepts without getting bogged down by low-level implementation details. This simplifies problem-solving and enhances code readability.
2) Code Organization:
- Definition: How code is structured and organized to achieve modularity and maintainability.
- Role: Programming paradigms guide programmers in organizing code into logical units, whether they are functions, objects, or modules. This aids in creating modular and reusable code, making it easier to understand and maintain.
3) Problem-Solving Approach:
- Definition: The method and mindset used to address problems and implement solutions.
- Role: Different programming paradigms offer distinct problem-solving approaches. For example, imperative paradigms focus on describing the steps to solve a problem, while declarative paradigms emphasize expressing what the solution should achieve. The choice of paradigm influences the overall approach to tackling coding challenges.
4) Flexibility and Expressiveness:
- Definition: The capability of a programming paradigm to adapt different types of problems and express solutions concisely.
- Role: Programming paradigms provide varying levels of flexibility and expressiveness. For example, functional programming allows for concise and expressive code through higher-order functions and immutability, while object-oriented programming supports the creation of flexible and extensible systems through encapsulation and inheritance.
5) Scalability:
- Definition: The ability of software to handle increasing complexity and size.
- Role: Choosing the right programming paradigm contributes to the scalability of a system. For example, object-oriented programming (OOP) encourages the creation of modular and scalable code by organizing it around objects and their interactions.
6) Readability and Maintainability:
- Definition: This refers to how easy it is to read and understand the code, and how manageable it is to make changes.
- Role: Programming paradigms impact code readability and maintainability. Structured programming, for example, enhances readability by organizing code into well-defined structures, reducing the likelihood of errors and easing maintenance.
7) Paradigm Combinations:
- Definition: The ability to use multiple paradigms within the same program or project.
- Role: Many programming languages support multiple paradigms. This allows programmers to leverage the strengths of different paradigms in various parts of codebase, and choosing the most suitable approach for each specific task.
At this point, one may get confused in between the programming languages and the programming paradigms. So, let’s differentiate between these two.
What is the difference between a programming language and the programming paradigm?
It's essential to understand both concepts as they collectively shape the landscape of programming or software development, allowing the programmers to choose the most suitable language-paradigm combination for their specific needs. A programming language serves as a formal system with defined syntax and semantics, acting as a tool for instructing computers to execute specific tasks. Examples include Python, Java, C++, etc. On the other hand, a programming paradigm is a broader methodology or style that influences how programmers approach problem-solving and code organization. It encompasses principles and practices guiding the overall structure, data management, and algorithm design within the code.
So, a programming language provides the specific rules and vocabulary for writing code, while a programming paradigm offers a higher-level perspective on how that code should be structured and how problems should be approached.
Many of you may have question in mind regarding the most effective programming paradigm. So, let’s cater this query as well.
Which one is the most effective programming paradigm?
Each programming paradigm comes with its own strengths and weaknesses, and what might be considered effective in one context may not be as suitable in another. Imperative programming paradigms, such as procedural and object-oriented, offer a clear and step-by-step approach towards problem-solving while emphasizing control flow and state manipulation. On the other hand, declarative paradigms like functional and logical programming focus on expressing the desired outcome, promoting code clarity, and immutability. The effectiveness of a paradigm often depends on factors like project requirements, ease of maintenance, scalability, and the programmer’s familiarity with a particular style. In practice, many modern programming languages support a combination of paradigms, allowing programmers to choose the one that aligns best with the specific goals and constraints of their project.
So, determining the most effective programming paradigm is a subjective matter that largely depends on the nature of the problem at hand and the preferences of the programmer or development team but yes, there are 4 major programming paradigms which are most commonly used in the field.
Which are the 4 major programming paradigms?
The four major programming paradigms which are commonly used are procedural, structured, functional, and object-oriented programming. Procedural programming focuses on organizing code into procedures or routines, outlining step-by-step instructions. Structured programming enhances clarity and maintainability through well-defined structures like loops and conditionals. Functional programming treats computation as the evaluation of mathematical functions, emphasizing immutability and higher-order functions. Object-oriented programming organizes code into objects, encapsulating data and behavior, fostering modularity and code reuse. Each paradigm offers a unique approach to solving problems, and the choice among them depends on the nature of the task at hand and the programmer’s preferred coding style.
The Conclusion:
In conclusion, programming involves instructing computers using algorithms with the aim to solving real-life problems. Programming languages serve as a bridge between humans and computers. The blog explores Imperative and Declarative Programming, with subcategories like Procedural, Structured, Object-Oriented, Functional, and Logical Programming.
Imperative Programming focuses on step-by-step execution, demonstrated through JavaScript examples. Declarative Programming emphasizes expressing logic without specifying control flow. The importance of paradigms lies in abstraction, code organization, problem-solving, flexibility, scalability, and readability, maintainability, and paradigm combinations.
The blog clarifies the distinction between programming languages and paradigms, highlighting that languages provide rules, while paradigms guide overall methodology. It addresses the subjectivity of the most effective paradigm, pointing out the significance of project requirements and programmer preferences. The four major paradigms—procedural, structured, functional, and object-oriented—offer distinct approaches based on the nature of the problem and coding style.
At last, if you really liked the blog and you find it helpful for you in any way, then do not forget to hit a like and comment below your valuable thoughts. This will help the writers at Discovering CS a lot. Thank You!
Comments by Readers:
Taimoor Bin Zahid Othmani
Very informative and brief article, very easy to understand for beginners and for those who want a quick understanding about programming paradigms.
Thank you so much for your kind words! We're really glad to hear that you found the article informative and easy to grasp, especially for beginners. It's always our goal to make complex topics accessible, so it's great to know that it resonated with you.
Suleman
I just finished reading the blog and it's really informative! The blog covers a wide range of topics in computer science, from algorithms and data structures to programming languages and artificial intelligence. The explanations are clear and easy to understand, making it a great resource for anyone interested in learning more about computer science. I particularly enjoyed the practical examples and interactive exercises that help reinforce the concepts. Overall, I think it's a fantastic blog for anyone looking to dive deeper into the world of computer science